devlog
Way more detail than you ever wanted to know about the development of the Anukari 3D Physics SynthesizerCaptain's Log: Stardate 78247.8
Having run into some more bugs with Filament, today I spent some time getting Filament set up as a git submodule that builds as part of Anukari's overall CMake config (instead of linking against a binary release). This has a number of advantages, the main one being that I can easily make local Filament modifications for debugging and bugfixes. Also, I can use the latest commits instead of the N-week-old release that the Filament folks produce. It does have the drawback of dealing with building Filament, but it wasn't too much of a problem (only took one PR to get it building).
Before digging into the Filament bugs I've seen, I wanted to get the 3D renderer preferences menu to a good stopping place. Namely, I wanted it to expose all the options, and I wanted low/med/high presets. I also had to implement a bit of a dependency system so that if, for example, Post-Processing is disabled, some of the other effects like FXAA need to be marked invisible or greyed out or whatever, since they do not work without Post-Processing.
This is all done, and it's really nice to be able to edit these parameters and see the results in realtime in the 3D editor window. And the "Max Speed" preset really is quite fast. I am pretty certain that it is no slower than my previous hand-rolled OpenGL implementation.
Next I will add something like a "3D Skin" tab, which to start off with will just contain a menu for changing the skybox. I have bought a number of commercial skybox models that I'll include with Anukari, and the user will be able to choose from those. In addition, I plan to allow custom skyboxes to be used. For now, this will be a power user feature since the user will need to run Filament's cmgen tool to convert their HDRI or EXR file into Anukari's format. Maybe eventually Anukari will do that itself, but it doesn't seem super important.
The 3D Skin tab will later include things for changing the 3D models for the Anukari objects, but I suspect that I will leave that until another time, and focus on stability with the new effects/skin tabs and cut a pre-alpha release as soon as practical.
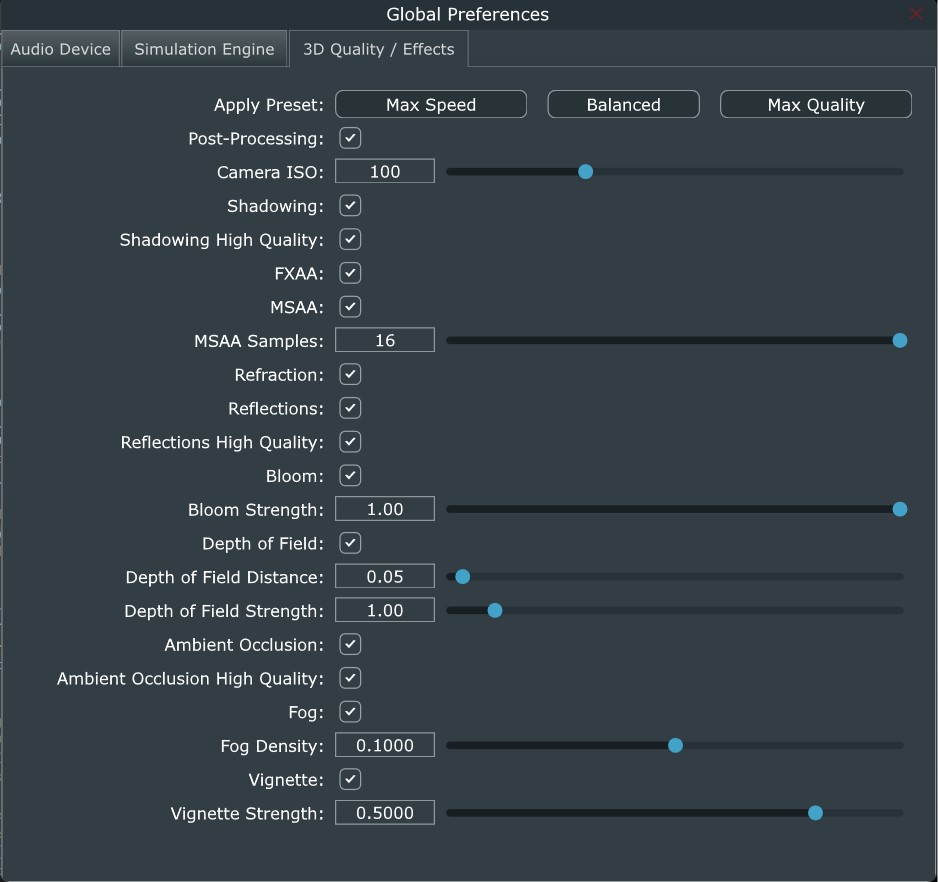
Captain's Log: Stardate 78245
MacOS Mouse Hover
Continuing my work from my previous devlog entry, I finally tracked down exactly what was going wrong with mouse hover on MacOS for floating native child windows. Basically there is a bug in JUCE where it allows mouse events to be duplicated between the frontmost child NSView and whatever NSView is behind it. In practice the mouseMove events were being sort of interleaved, so JUCE saw the mouse as entering/exiting the button and then entering/exiting the window below the button on each event.
The fix was pretty simple. Here's hoping that the JUCE folks will accept my pull request.
OpenGL on Intel Iris Xe Chips
In the previous devlog entry I was investigating Google Filament crashes with the Vulkan backend. While doing that, I figured I'd just change the default backend to OpenGL to get things working in the meantime. That worked great on my NVIDIA desktop chip, but on my Intel Iris XE chip OpenGL displays a black screen.
I haven't tracked down the root cause here yet, but I debugged it to a pretty significant extent and am hoping to hand off further debug work to the Filament team in this bug.
While working on this, I found other issues with Filament, like the fact that it crashes in debug mode on MSVC and in general misuses its own panic feature. I have a feeling the Filament folks are going to be very annoyed with me; I've already discovered more bugs that I haven't yet filed.
Note: anyone who wants Anukari to succeed might like to add smiley emojis on all of these Github issues to indicate that they're important.
Configurable Graphics Settings
After fighting with all these third-party library bugs, I finally had a little bit of time today to spend on fun stuff, namely: making the graphics quality settings fully-configurable. Google Filament has a lot of options, and I plan to expose most of them to the user. Right now I have the majority of the options configurable via the preferences menu system, and it's been a blast to play around with different settings. The screenshots below show some fancy stuff you can do with bloom, depth-of-field, and camera ISO. There are also options for fog, various quality things like FXAA and MSAA, and everything can be disabled or turned to low-quality. Once I'm done with the preferences menu, it will be possible to turn the graphics down to the point that they're faster than the old renderer, while still looking much nicer.
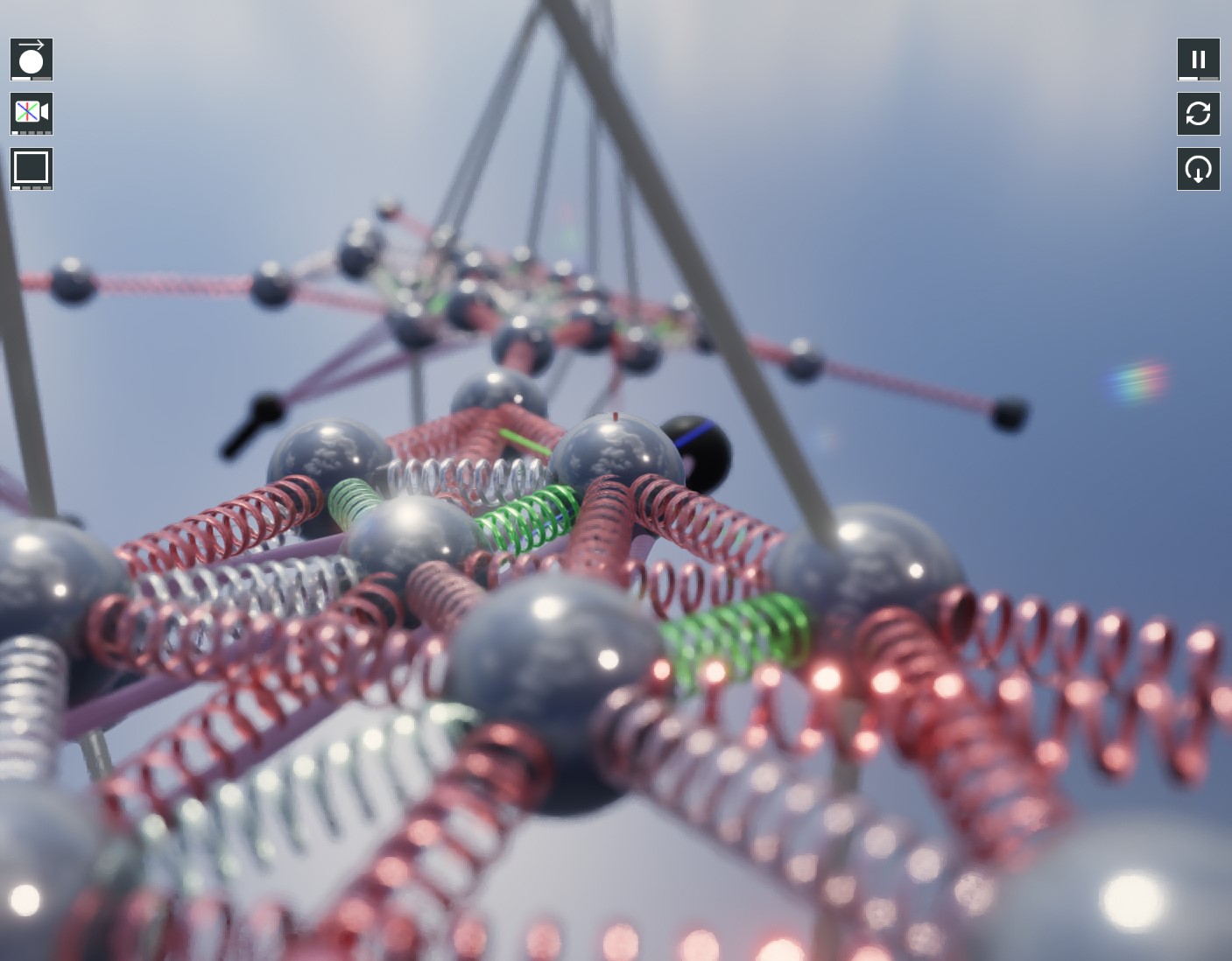
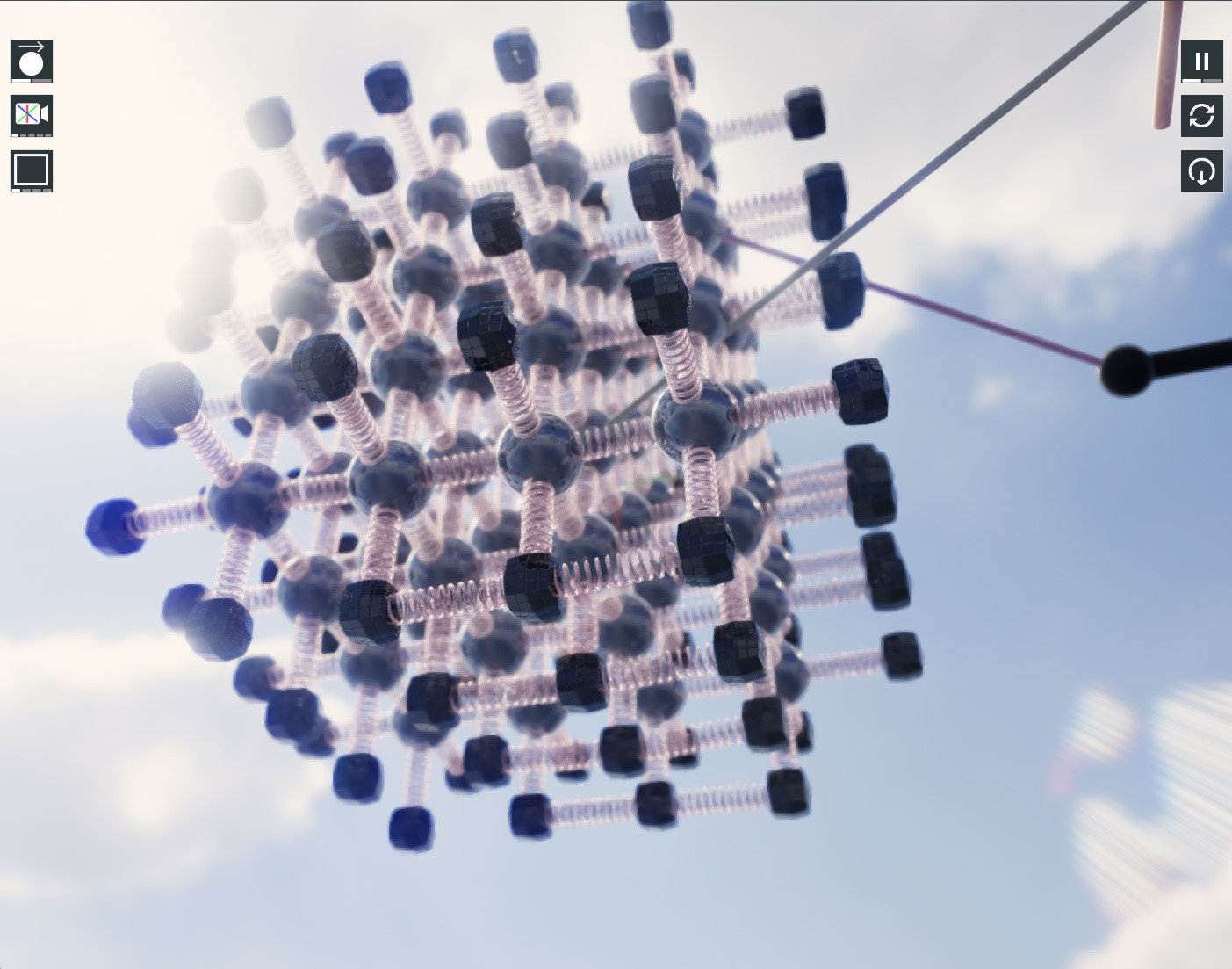
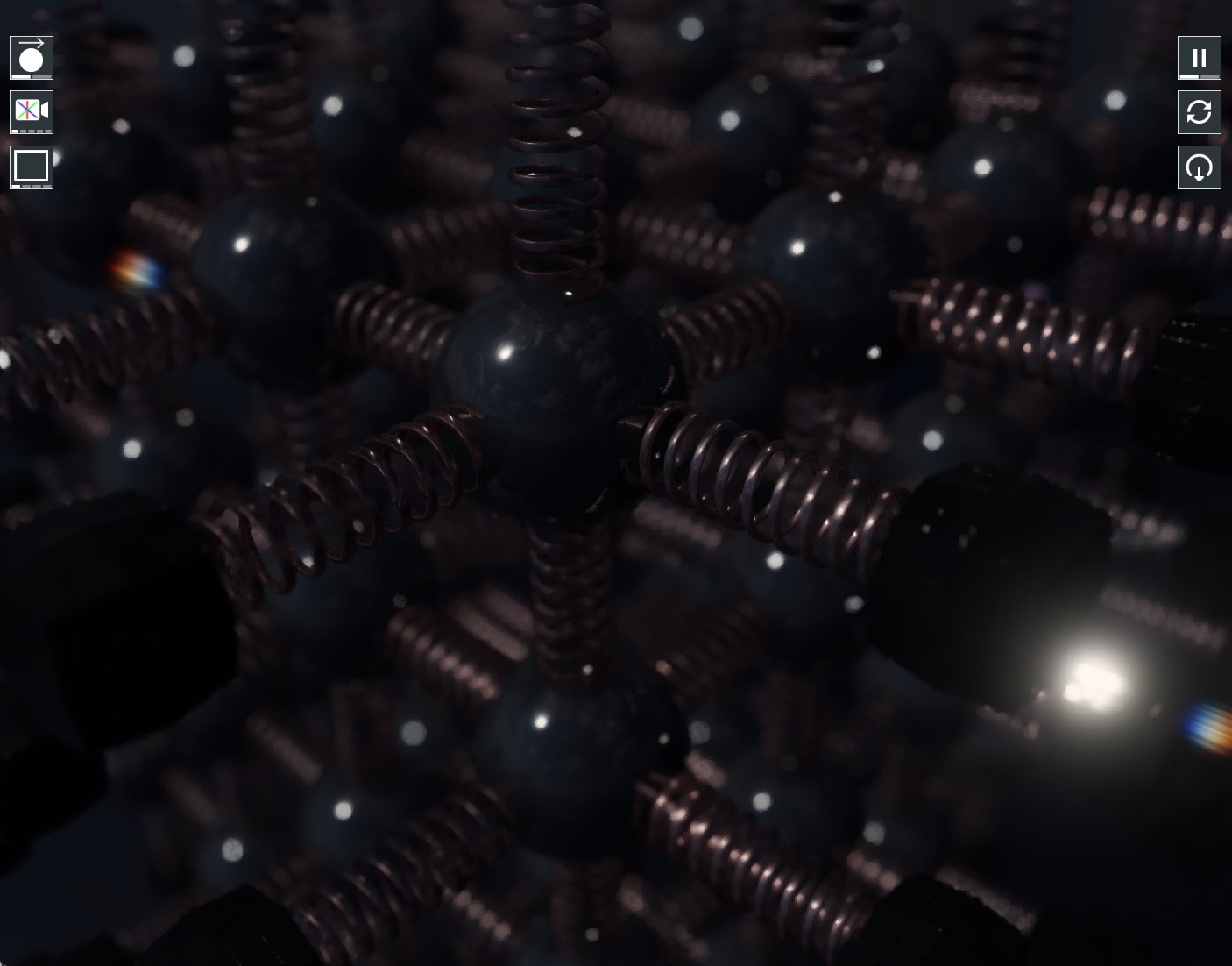
Captain's Log: Stardate 78236.8
Over the last couple of days I've been trying to track down some problems with the latest pre-alpha build.
Resizing Crashes
A user reported that resizing the window caused crashes on the Vulkan renderer, which was weird because I had tested that and never saw any issues. This user was on a laptop with an Intel Iris Xe GPU, though, which immediately made me suspicious since the Intel drivers have a tendency to suck. Fortunately I have a laptop with this chip, and was able to repro the issue right away.
After attaching a debugger and resizing the window, my machine didn't crash but did hang. I found that the hang was somewhere deep in Google Filament's Vulkan code, but it was pretty unclear what it was doing. Filament currently has a bug that prevents it from running in debug builds under MSVC, so I didn't have full symbol information.
I got suspicious, though, because the problem didn't seem like anything I was doing. So I ran Anukari on my main machine with an NVIDIA chip, and resized the window like crazy. Eventually it crashed. Given that it crashed quickly on the Intel chip, and took a long time on the NVIDIA chip, I guessed that maybe this was a resource exhaustion issue. Fortunately on the NVIDIA machine it didn't hang, and I got a Filament panic message about not being able to create a new swap chain.
I read the Filament code a bunch and eventually came to the conclusion that when the window is resized, and a new swap chain is created, the old one is never destroyed via vkDestroySwapchainKHR(). Thus it is leaked and we're guaranteed to eventually crash. I filed this bug.
As to why the Filament authors failed to call the destroy function, I ran into this Vulkan bug. It turns out that it's super unclear when it is safe to destroy a swap chain, to the extent that they had to add an extension to the spec to make it safe to do.
MacOS Mouse Hover
I thought to myself, well, at least things work well on MacOS. I was messing with the GUI on Mac today and discovered that tooltips weren't appearing for the buttons that hover over the 3D view. Furthermore, the hover states for those elements weren't working properly. When you mouse over them, you see the hover state for a split second but it doesn't stay. I found that mouse exit events were firing before the mouse left the window.
Initially I assumed that this had to do with my weird CAMetalLayer-backed NSView for the 3D rendering, but the issue still happens if I don't create the renderer window. I started adding instrumentation to JUCE's NSView event handling, and MacOS seems to be calling into it in a perfectly sane way -- mouseEnter and mouseExit call when I think they should.
I'm still not totally clear what's going wrong, but I have narrowed it down to the fact that JUCE is getting confused about which window the mouse is over: the child window, or the parent window that's obscured by the child window. So it alternates back and forth between the two juce::Components on each mouseMove event, and when it sees the current Component change it generates a mouseExit for the previous one.
Obviously there's a problem with how JUCE is handling the fact that the child window obscures the parent window, but I'm not yet sure how.